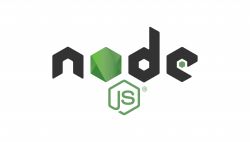
Recently, I was running a simple system for reporting energy measurements via a WiFi network from a "smart" electrical outlet and I needed a convenient way to test the reception of HTTP GET and POST requests. I will show here how you can use Node.js for this purpose. Node.js allows you to quickly and efficiently set up a mini-HTTP server on our machine, capable of processing these queries and responding to them in the way we specify. By the way, I will also show how you can handle a POST query with content in JSON format and I will put here short examples of sending GET and POST from the OpenBeken
NOTE: The topic assumes that the reader has at least minimal knowledge of how to use the console, how to install Node.js, what is the server, HTTP, and so on...
Sending HTTP requests
In this topic, I assume that we already have the code for sending requests (i.e. the client), and we need to create a mini server for testing, but it is still worth highlighting how these requests can be sent.
For example, you can use a library curl which is available on multiple platforms:
https://curl.se/
Another way may be to send directly from Javascript, even if executed in the browser, we can create an HTML file and play with it XMLHttpRequest be fetch and so on.
Yet another, extremely complicated way is to send queries from your own environment, for example, at my place OpenBeken there are functions to send GET and POST, these are the functions I tested when creating this theme.
Regardless of the choice of the client, in this topic I will show you how to create a simple HTTP server in Node.js, capable of receiving the mentioned GET and POST.
HTTP GET support
The GET query is probably the most popular on the web, it allows you to encode arguments in the URL of the page itself, even on our forum when writing a post we see:
https://www.elektroda.pl/rtvforum/posting.php?mode=reply&t=3977701&p=20578056
The GET arguments are after the question mark, we can see here that the "mode" key is "reply" and the "t" key is "3977701", etc.
As an example, we'll make a simple calculator. As arguments will be a and b, the server will return their sum to us. We create the server.js file and save:
Code: Javascript
Calling app.get in the code above is not sending a GET request, but creating a callback that will handle it. In it we receive and process data. By req.query we're getting the arguments from the URL.
We navigate in CMD to the folder where the script is, and run it via nodeserver.js , but we will probably get an error - there is no package express , you need to install it via npm install express here is the whole log:
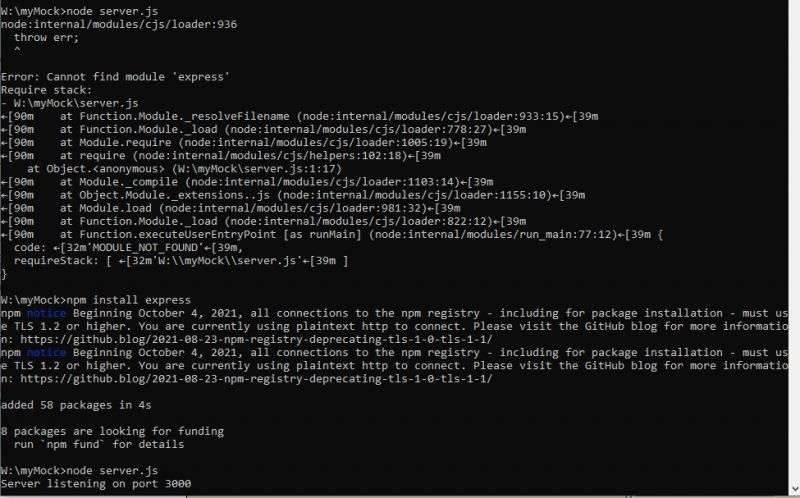
Then already nodeserver.js is performed correctly.
Now you need to somehow send data to it , method one - browser, open the URL:
http://127.0.0.1:3000/?a=13&b=87
Works:
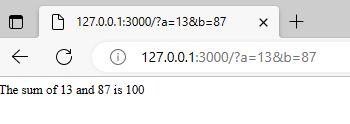
In the server log we also see that something is going on:

Now let's send a GET request via CURL:
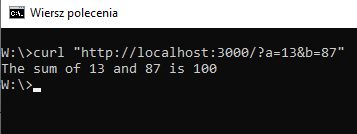
Of course, CURL must be installed beforehand.
And as a curiosity - the same with OpenBeken:
backlog setChannel 10 13; setChannel 11 87; SendGET http://localhost:3000/?a=$CH10&b=$CH11
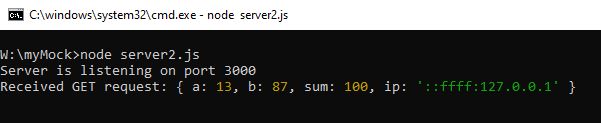
In OBK, this mechanism does not yet support parsing responses from the script level, it is only used to report data to the server.
HTTP POST support
Now it will be a bit more difficult, because the POST content is already contained in the body of the query, not in the URL, so it is basically invisible at first glance. Of course, POST also occurs even on our forum, for example, when we log in or register, then our login and password are sent with a POST query.
The post additionally introduces the so-called Content-Type , i.e. the type of query content, it can be, for example, plain text, binary stream or just JSON format.
What does JSON look like? Sample JSON below:
Code: JSON
JSON can store objects along with key-value pairs and arrays.
As an example, let's rewrite our code so that it uses JSON format but still performs the same operation.
Code: Javascript
As before, we created a callback in app.get , here we create the post service in the same way app.post . Now we take a and b from the text in JSON format, from the body of the query.
Now you have to test it. Let's start with the CURL method:
curl -X POST -H "Content-Type: application/json" -d "{\"a\": 13, \"b\": 87}" http://localhost:3000/
Switch X sets the query type, H adds a Content-Type header specifying what we send, -d sets the data. Quotation marks with \ are so-called escape sequence, allows you to put quotation marks in the body of the query without actually closing the quotation marks specifying the block of data.
Everything works:
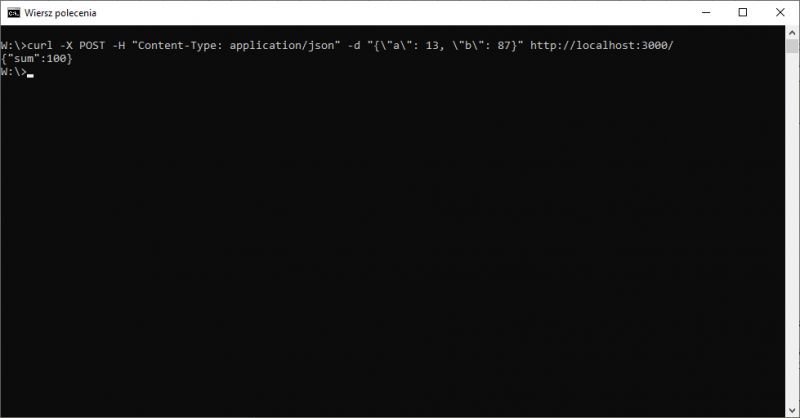
As an example, let's see how the same can be sent from Javascript itself - from a simple HTML file, run in the browser, without setting up a server. Anyone can easily fire it:
Code: HTML, XML
Unfortunately, this script will not execute correctly. There is a problem with CORS (Cross-Origin Resource Sharing) here.
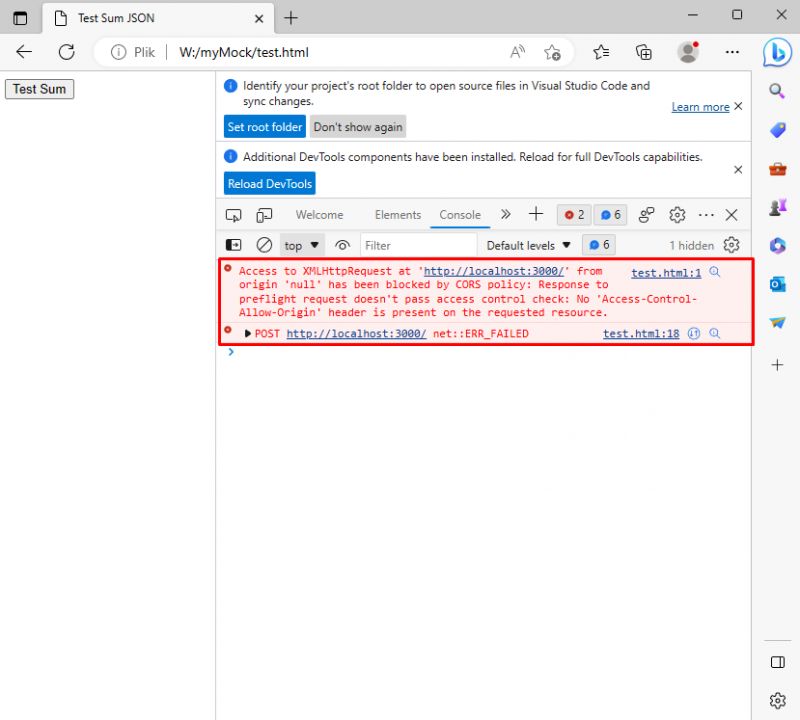
Error content as text:
p://localhost:3000/' from origin 'null' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource.
test.html:18
POST http://localhost:3000/ net::ERR_FAILED
sendSumRequest @ test.html:18
onclick @ test.html:23
CORS is blocking external Ajax requests to make resources more secure, we need to unblock them. We modify the server:
Code: Javascript
We are not firing yet, otherwise we would get an error:
Error: Cannot find module 'cors'
so we install the missing package:
npm install cors
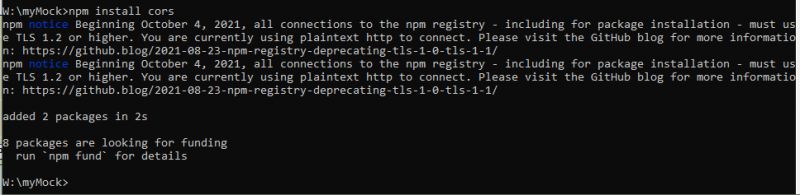
After this change (and server restart) the script works:
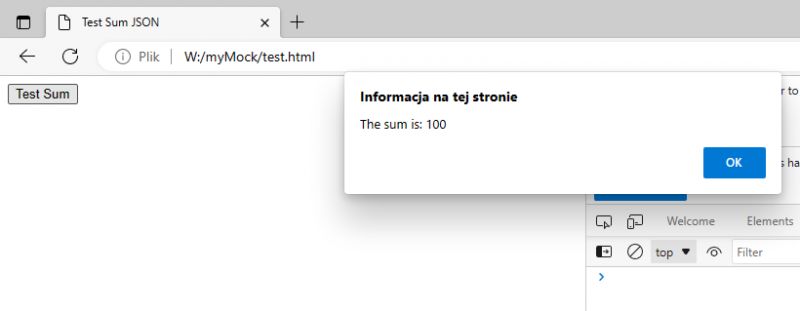
And as a curiosity, the same with OpenBeken:
backlog setChannel 10 13; setChannel 11 87; SendPOST http://localhost:3000/ 3000 "application/json" "{ \"a\":$CH10, \"b\":$CH11 }"
The code specifically, as part of the demonstration, sets the channels to 13 and 87 and then uses their values when generating JSON.
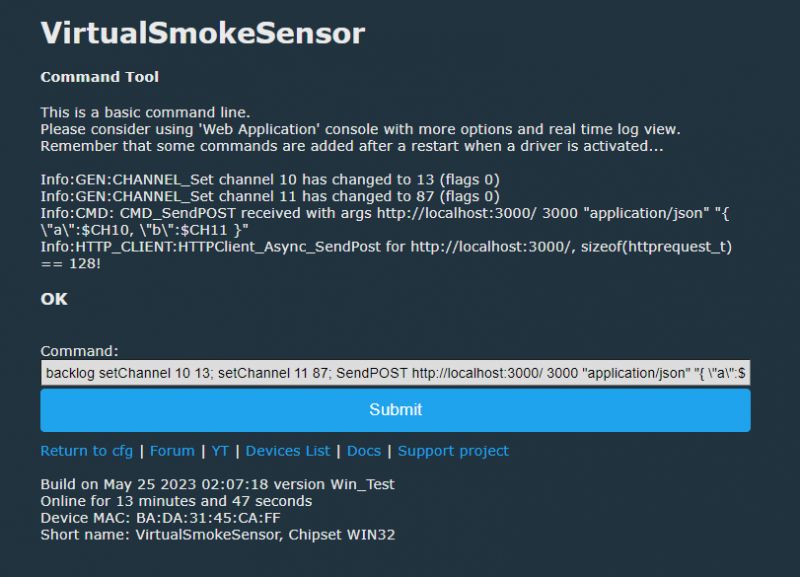
Result:
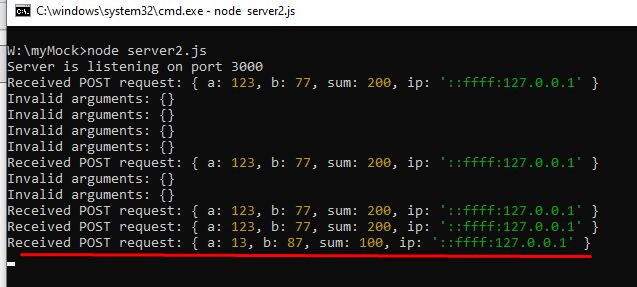
Summary
Node.js offers a very convenient collection of tools and libraries for building server-side applications in Javascript. Here I showed how they can be used to create a mini-server for testing the reception of GET and POST requests, because ultimately I intend to put the server on one of the free web hosting available on the web and the requests themselves will be received at the PHP level, but nothing stands in the way of use Node.js differently, all at your discretion.
In a similar way, you can also access a database directly, e.g. InfluxDB, by using their HTTP API:
https://archive.docs.influxdata.com/influxdb/v1.2/guides/writing_data/
Perhaps soon I will show a specific example of implementing a temperature or voltage/current/power logger based on the mechanisms shown here.
Cool? Ranking DIY Helpful post? Buy me a coffee.